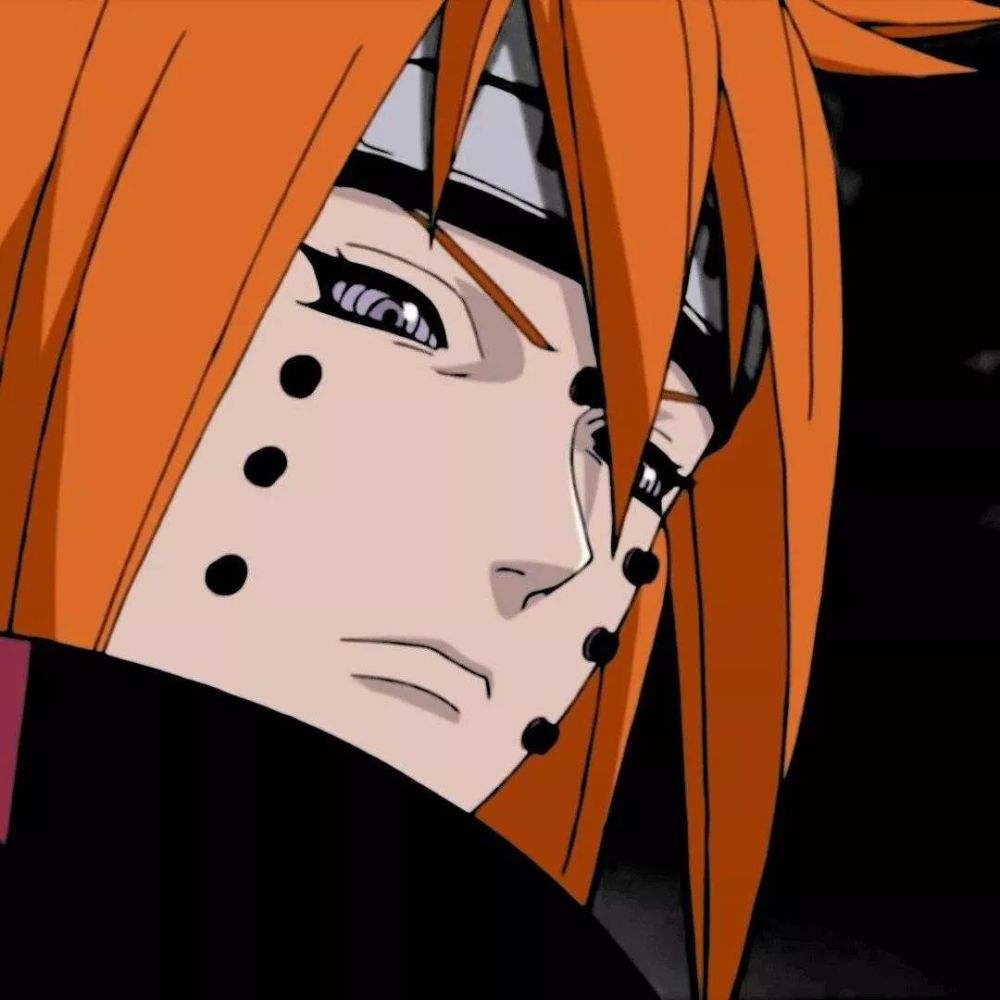
1. Skywalking
1.1 Segment
Segment是一批span的集合,它表示一个线程上下文,如果跨线程则需要重新记录为一个segment;跨进程可以表示发起了一个跨线程调用,更多地情况(也是链路追踪最大的意义)是表示了两个服务之间的调用。
- traceId:一条调用链路的唯一id,用其串联起一条链路
- traceSegmentId:一个segment的id
- spans:该segment上下文中所有的span
- service:表示该segment所在的服务
- serviceInstance:表示该segment所在的服务实例(在kubernetes中对应一个pod,服务基本都是多实例部署的)
- isSizeLimited:是否有一些span因为过大被丢弃了
1 | message SegmentObject { |
1.2 Span
span是segment下层的概念,它的含义是一个更细粒度的方法调用
spanId:该span的id,从0开始,在一个segment中保证唯一
parentSpanId:该span在这个segment中的父亲span的id,如果是-1表示没有父亲span,则该span是根span
startTime:开始时间
endTime:结束时间,开始时间和结束时间可以得到耗时信息
refs:如果发生了跨线程/进程,记录上个segment的信息;如果是MQ或者批量操作的情况下,会有多个ref
operationName:span的操作名称
peer:如果是exit span,记录要调用的接口或http请求
spanType:
- entry span:一个segment的头部span
- local span:一个segment本地操作的span
- exit span:一个segment的尾部span,标志一个跨线程调用的发起
spanLayer:span所在的服务的类型,rpc,db,cache等
componentId:预定义的id,根据埋点的组件决定,比如spring等
isError:该span是否错误
tags:键值对,给span打标签
logs:记录该span中发生的事件,记录键值对和时间
skipAnalysis:是否跳过分析
1 | message SpanObject { |
1.2.1 SpanType
1 | // Map to the type of span |
1.2.2 SpanLayer
1 | // Map to the layer of span |
1.2.3 Log
1 | message Log { |
1.3 Segment Reference
segment reference用于连接两个segment,把他们串起来。使用segment reference可以根据服务侧的entry span方便地生成拓扑,具体拓扑生成的方式可以看wusheng的这篇STAM分析方法。
- refType:父亲segment到儿子segment的调用关系,是跨进程还是跨线程
- traceId:父亲segment的trace id
- parentTraceSegmentId:父亲segment的segment id
- parentSpanId:父亲segment的exit span id
- parentService:父亲segment的服务名称
- parentServiceInstance:父亲segment的服务实例
- parentEndpoint:父亲segment的enpoint信息,如果父亲服务是http服务,那么就是一个父亲服务的url;如果父亲服务是rpc服务,那么就是它的服务名+接口。这个也对应父亲segment的entry span的operation name。
- networkAddressUsedAtPeer:客户端调用服务端,服务端的网络地址;这个等同于exit span的peer
1 | message SegmentReference { |
2. OpenTelemetry
其实总的来说链路追踪的协议设计上都比较接近,但是opentelemetry没有直接的segment的概念
2.1 一批trace数据的集合
1 | message TracesData { |
2.2 对应一条trace的概念
1 | // A collection of ScopeSpans from a Resource. |
2.3 对应一个segment
1 | // A collection of Spans produced by an InstrumentationScope. |
2.4 基本单元,Span
- trace_id
- span_id
- trace_state:它用于在分布式跟踪的环境中传递有关当前 Trace 的数据,可以是键值对的形式组成string
- parent_span_id
- flags:标识位表示该span是否是远程调用
- name
- kind:span的类型,不过使用了server、client、internal、producer、consumer和unspecified
- start_time_unix_nano
- end_time_unix_nano
- attributes:属性键值对,对当前span打了标签
- dropped_attributes_count
- events:更细粒度的事件
- dropped_events_count
- links:用于将span进行串联
- dropped_links_count
- status:span的状态
1 | // A Span represents a single operation performed by a single component of the system. |
2.4.1 SpanKind
表示span的类型,不同于skywalking中的entry span、local span和exit span,其使用internal对应了local span,使用server、client表示了调用关系的entry span和exit span,但是其特别标识了不明确类型的span,以及区分出了消费者和生产者的span,对应于MQ的场景。
1 | // SpanKind is the type of span. Can be used to specify additional relationships between spans |
2.4.2 Event
是对更细粒度的操作的记录,比如一个Rpc操作可以用Event记录调用下游的ip、zone是什么,以及操作类型等
1 | // Event is a time-stamped annotation of the span, consisting of user-supplied |
2.4.3 Link
相当于skywalking中segment reference的概念,可以将服务等信息存在键值对结构的attributes中,表示了span之间的调用、串联关系
1 | // A pointer from the current span to another span in the same trace or in a |
2.4.4 Status
用于表示Span的状态,skywalking中直接用isError表示为是否是错误的
1 | message Status { |
2.4.5 Span Flag
标识位表示该span是否是远程调用
1 | enum SpanFlags { |
3. Xray
- 本文标题:链路追踪常见协议实现
- 本文作者:mufiye
- 创建时间:2024-09-20 23:21:19
- 本文链接:http://mufiye.github.io/2024/09/20/链路追踪常见协议实现/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!