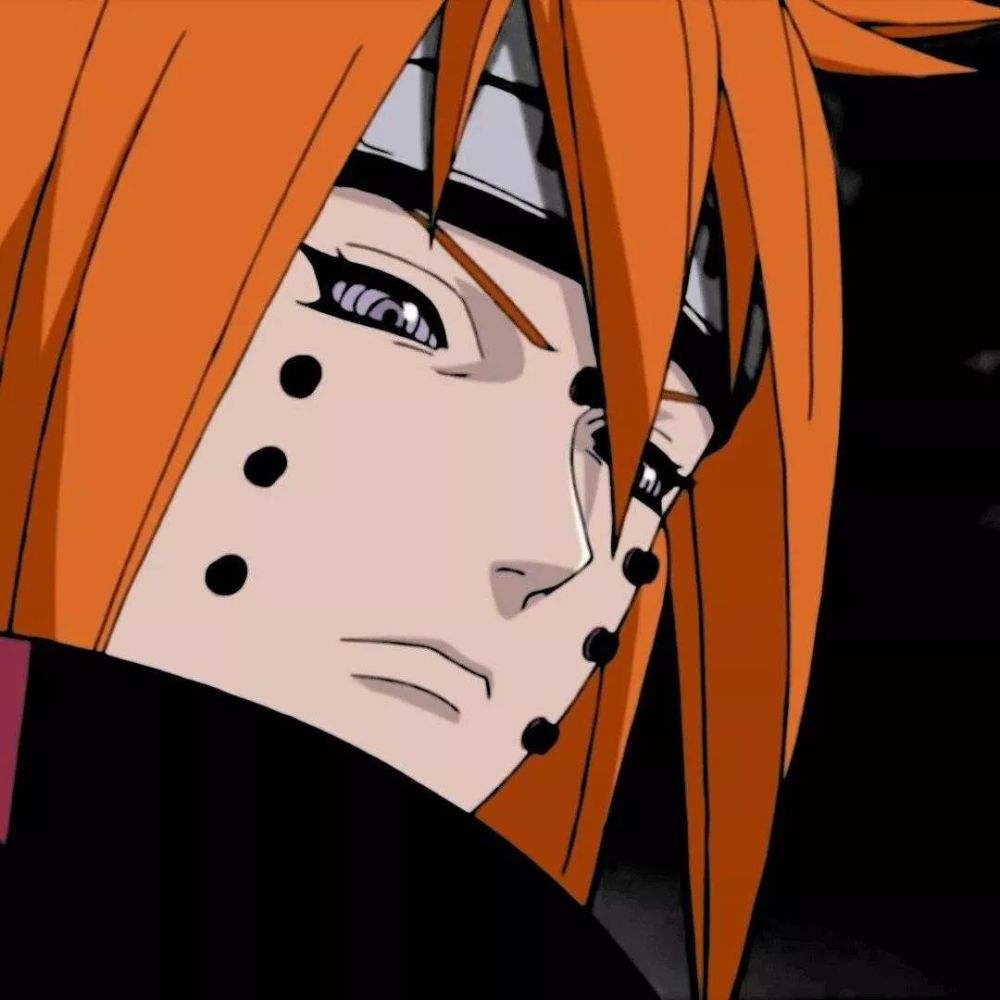
n皇后问题
一、问题介绍
n皇后问题借鉴了国际象棋中皇后的概念,此处我先介绍一下这里的皇后指的是什么。在国际象棋中,在一个棋盘上,皇后可以横着走、竖着走和斜着走。而n皇后问题指的就是,假设有n个皇后,在一张n*n的棋盘上,如何将这些皇后放在这张棋盘上,是这些皇后彼此不在对方的打击范围内,也就是怎么样放才能使她们都是安全的。
二、算法
在本文中,我将使用回溯算法来解决这个问题。回溯算法实际上一个类似枚举的搜索尝试过程,主要是在搜索尝试过程中寻找问题的解,当发现已不满足求解条件时,就“回溯”返回,尝试别的路径。用回溯算法解决问题的一般步骤一是针对所给问题,定义问题的解空间,二是确定易于搜索的解空间结构,使得能用回溯法方便地搜索整个解空间,三是以深度优先的方式搜索解空间,并且在搜索过程中用剪枝函数避免无效搜索。
针对n皇后问题,我们可以将棋盘表示为一个n行n列的矩阵,我们将这个矩阵初始化为一个全0的矩阵,假如这个棋盘的i行j列放了一个皇后,我们就将这个矩阵的i行j列的值设置为1。由题设,我们易知每一行有且只有一个皇后,我们可以用一个树的结构来表示整个搜索过程,因此我们可以将每一层树定义为将每一行的皇后放入棋盘中的某一列。之后我们进行深度优先搜索,如果某一次将皇后放入棋盘导致一些皇后不安全,那么我们就把这一次放置的情况作为根的树剪掉,并且回溯到之前的放法,直到遍历完整颗树。
三、代码实现
为了节省资源,我发现不用使用空间复杂度过大的矩阵而使用向量也能够表示将皇后放在棋盘的某个位置并完成搜索。因为为了保证每个皇后的安全,每一行有且只有一个皇后,那么我们可以用向量的第一个元素表示第一行的皇后放在哪一列,第二个元素表示第二行的皇后放在哪一列,第n个元素表示第n行的皇后放在哪一列。
在具体的代码中,我使用了递归函数solveNQ来实现搜索,使用is_safe来判断放入某个皇后是否会导致某些皇后不安全,最后我定义了一个findAllNQ函数来实现保证搜索完了整一棵树,得到所有的解。以下为具体的代码及测试结果:
代码:
1 | def findAllNQ(n,solution): |
测试1:
1 | solution=[] |
结果1:
1 | ------------------------- |
测试2:
1 | solution=[] |
结果2:
1 | ------------------------- |
四、结论
在本文中,我成功使用回溯算法解决了n皇后问题,回溯算法作为一种特殊的穷举算法,使用深度优先搜索和剪枝大大降低了算法复杂度,而在解决n皇后问题时,使用向量代替矩阵来表示解空间又大大降低了解决该问题的算法复杂度。
- 本文标题:n皇后问题
- 本文作者:mufiye
- 创建时间:2021-05-13 11:40:57
- 本文链接:http://mufiye.github.io/2021/05/13/n皇后问题/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!